Speech Recognition Using Python: Convert Speech to text and text to Speech
Speech recognition is an important feature in many applications used such as home automation, artificial intelligence, etc. This article is an introduction to using the Python SpeechRecognition library.
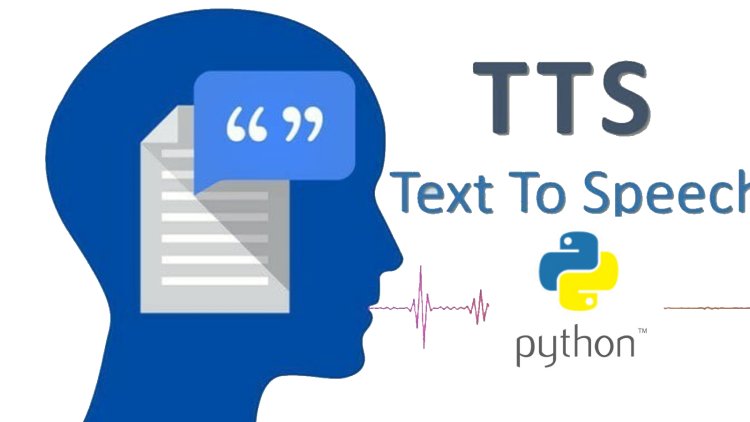
Speech recognition is an important feature in many applications used such as home automation, artificial intelligence, etc. This article is an introduction to using the Python SpeechRecognition library and pyttsx3.
Installation required:
-
Python Speech Recognition module:
pip install speechrecognition
-
PyAudio:
Use the following command for linux os
sudo apt-get install python3-pyaudio
Windows users can install pyaudio by executing the following command in a terminal
pip install pyaudio
- Python pyttsx3 module:
pip install pyttsx3
Microphone voice input and Speech-to-Text :
Allow adjustment for ambient noise.
As the ambient noise changes, the program should be given a second or too long to adjust the recording energy threshold to adjust to external noise levels.
Speech to text: This is done using Google Speech Recognition. An active internet connection is required to work.
However, while there are certain standalone recognition systems like PocketSphinx, they require a very strict installation process that requires multiple dependencies.
Google Speech Recognition is one of the easiest to use.
Pyhton Program for Speech to text:
# Python program to translate
import
speech_recognition as sr
import
pyttsx3
r
=
sr.Recognizer()
def
SpeakText(command):
engine
=
pyttsx3.init()
engine.say(command)
engine.runAndWait()
while
(
1
):
try
:
with sr.Microphone() as source2:
r.adjust_for_ambient_noise(source2, duration
=
0.2
)
audio2
=
r.listen(source2)
MyText
=
r.recognize_google(audio2)
MyText
=
MyText.lower()
print
(
"Did you say "
+
MyText)
SpeakText(MyText)
except
sr.RequestError as e:
print
(
"Could not request results; {0}"
.
format
(e))
except
sr.UnknownValueError:
print
(
"unknown error occured"
)
|